Top 10 React Libraries Every JavaScript Professional Should Know
React is one of the three most popular solutions used by front-end developers. According to NPM’s statistics it has the biggest number of downloads. Unlike the other two solutions (Vue and Angular) React is not a full-blown framework but rather a library for building UIs, so if you plan to develop a more advanced project, you will probably need to pair React with some additional libraries. There are a lot to choose from, so it would be nice to narrow it down a little bit. In this article, you will learn about our top 10 React libraries.
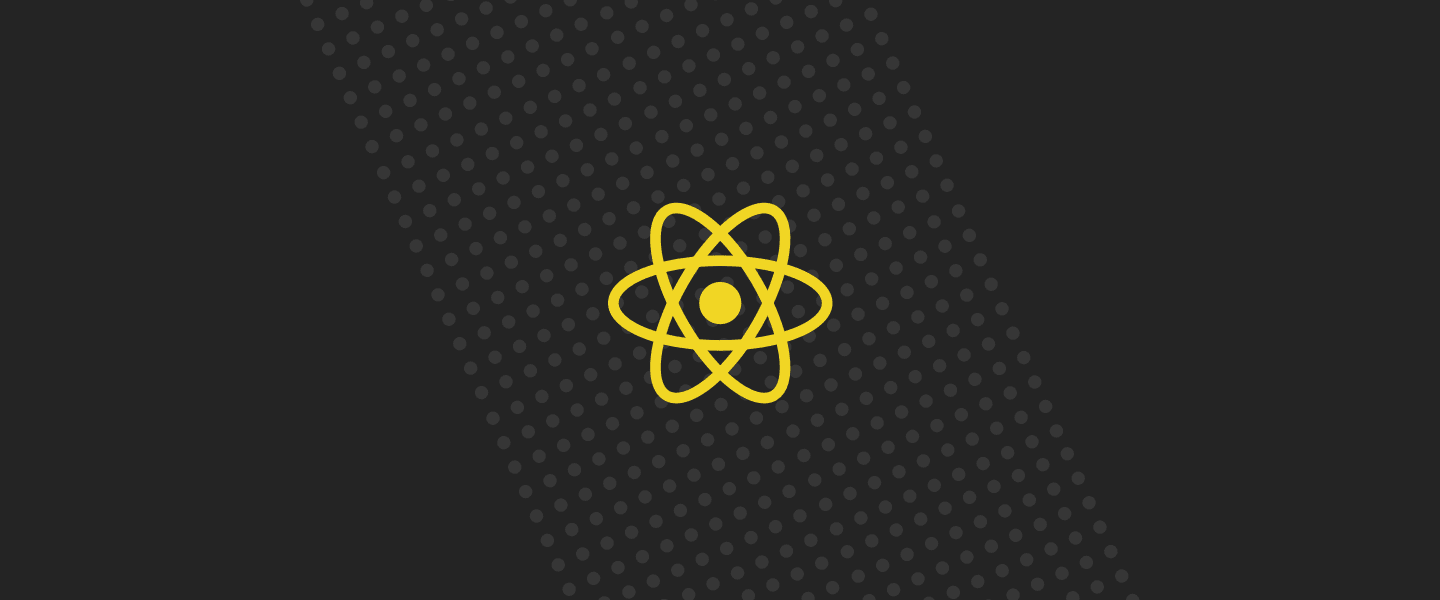
Table of contents
Redux, state management for your React apps
Do you remember the times when you had 10 flash drives, each with different data, lying around your house? That’s similar to pure React apps with components only having their own local states. In some cases that’s enough (mostly in small apps where data doesn’t need to be shared), but in larger projects, you want to have a centralized place for your data.
Imagine that we put those flash drives in the cloud where everyone can reach them. That’s what Redux is like. Brilliant, right? The data can be shared between all the app’s components and updated using a set of predefined actions and pure functions (reducers). This mechanism is predictable, which means that with the same actions in the same order we’ll always end up with the same state, and that makes it easy to test and debug. It’s also flexible, you don’t have to go all the way, you can use both the component’s state and redux store in one project.
Enzyme, a great way to test react components
This one is very common in day-to-day life. Consider other things that you use every day: a computer, a car, a smartphone. All of them need to be certified to confirm they’re safe to use and they function properly. To provide that kind of reliability, you need a team of experts to put these devices through rigorous tests.
Enzyme is a tool that can help software specialists test their React components. It’s compatible with many popular test runners (e.g. Mocha, Jest, Jasmine), so setting it up won’t be an issue. Now, you can rest assured that the core parts of your application are well written.
Redux Form for building safe forms in React
You’ve probably signed legal documents many times in your life. Usually a signature from both parties is enough to seal a deal. Sometimes however, when big money is on the table, you ask for a signature in the presence of a notary, who acts as an external, neutral party and registers the deal.
This special precaution is also crucial in the applications world. Identity theft can be a serious problem when it comes to online transactions. Hackers can easily distort online forms in HTML & CSS. The solution to this was found a long time ago and is implemented in Redux Form. This library prevents attackers from injecting additional data into forms, or even submission of such forms without user notice.
All of these prevention mechanisms are bundled into the Redux Form solution. With this React library you can build not only safe but also beautiful material design forms. On the documentation page you can find many examples of how to create a specific form and add custom validation rules.
Eslint, an open source library to keep your code clean
Programming is similar to writing a book. To create a novel you need to do at least some of these tasks: planning, writing, checking, editing and publishing. Let’s focus on proofreader checking. Without it your book would surely be full of errors. If you have been using Microsoft Word, you’ve noticed that it has built-in AutoCorrect function. With this feature, you can get rid of spelling and grammar mistakes.
The same responsibility lies with a linter. Eslint checks syntax and suggests best practices. You can use default rules or configure one of the popular style guides, e.g. Google or AirBnB. It can also be configured to run in a CI environment and protect from the dangers of merging new pieces of code if any errors occur. Of course not everything can be verified by a linter. You should definitely use one, but don’t expect that this alone will make your code great. When it comes to the solution review and code patterns, you should always rely on a good old code review by a peer developer
Material UI implements material design for your react components
Nowadays, almost everyone has a decent camera built-in to their smartphones, but this fact doesn’t make us professionals. Experienced photographers and programmers have a lot in common - even though they can create their work from scratch, they often use ready-made solutions to optimize the process. It’s hard to imagine a professional photographer without reflectors, lighting accessories or photo editing tools.
Similarly, developers have their own tools to turn code into great looking applications. Material UI helps to create a consistent look for an app by using building blocks with ready-to-use components. All elements have been tested and are intuitive, which has a positive impact on user experience. You can also use the built-in color palette and enjoy the fact that all components implement responsive web design out of the box. Using them can save many hours of work.
React Intl - support for multi language applications
This one is pretty straightforward: you want to make your content available for people all over the world, you need to help them understand it. This library makes it quite simple for developers to make that happen in your app! Your users will feel comfortable seeing the content formatted and translated according to the standards they are used to.
Not only does it support translations for 150+ languages but also helps with pluralization of nouns, which varies from country to country. For example in English we have two forms:
but in Polish we have four of them:
React Intl takes care of that for you. All you have to do is specify the translations for particular numeric values and pass them to the component.
This awesome library also supports different date and time formats. You can easily present the date in this form: 3/20/2019 10:29; or in this one: March 20, 2019; 10:29 AM. The same goes for different number formats, e.g. 1,234.56 (US) and 1 234.56 (France).
When it comes to internationalization of your app, React Intl is a way to go!
Storybook, an environment for ui components
The moment of unboxing a new purchase is a spectacular one. For many people, this event in itself is fun, quite aside from the item being unpacked. Industry experts know this and often work to make even unboxing a unique experience for the user. Take Apple products and their brilliant design as an example. Unpacking a new iPhone is a beautiful event learn from them.
Make the process of onboarding new developers simple and easy. This experience is strongly tied to one exceptional library: Storybook. The library enables developers to create a book of usages for features. You not only see what the components look like, but also how they behave. This pays off in the long term as new developers can get a glimpse of usage in a matter of seconds. This saves an enormous amount of time and helps in collaborations. Nowadays, this is a must in a mature team.
Reach Router simple way to build routes
Do you remember the old-school helpdesk and support lines? You dialed one number, to get redirected to another, and after 10 minutes, a final redirect to start the goddamn case. Frustrating. An automated helpline should have questions constructed in such a way that it can be quickly and intuitively accessed by the user to answer their question.
Similarly for the user experience on your website: the important bits should be within reach or not further than two clicks away. Thus, you need a way to organize things. Instead of one entry place with all information, you should create many: for landings, for user management, for documentation, etc. To create modern website addresses like www.yourbrand.com/myproduct/functionality you need a solution like Reach Router, a shiny successor to the oldie React Router that took the community by storm. Same author, better capabilities.
GraphQL is great for fetching data
In the past, traditional post offices were more popular and sending a parcel took a few days. Currently, almost every major transport company offers “same-day delivery” service. This amazing possibility is a result of brilliant process optimization.
Fast data transfer is crucial in creating web applications. The “packages” that your app delivers are chunks of data stored in your database. To get them fast to the end user you need an API and a transportation layer. Over the years developing these has usually taken a great amount of time and resulted in front-end developers’ work depending on the backend. Luckily for you, GraphQL is a great architecture that aims to solve the problem and deliver data as fast as possible. Also, in contrast to the REST API, it gives you more flexibility when it comes to getting a specific piece of information.
Gatsby, a popular framework that speeds up React applications
Let’s go back to cameras now - polaroids, specifically. They can be useful at a meeting with friends to let you capture unforgettable moments. Once you’ve taken the photos you have to wait some time for them to develop, but when they do you have a set of freshly-made, fully static representations of the dynamic stuff that you started with.
It’s very similar in Gatsby, which is a Static Site Generator. Static resources are created from multiple static information providers like database information, markdown files, CMS configuration, etc. Instead of querying these providers over and over again, they are extracted as static assets. One command, gatsby build is enough to make an application ready for deployment. So easy! Gatsby provides great performance and security because you don’t need to communicate with the API.
Summary
Each of the libraries listed above has a different use: taking care of interfaces, user experience, application performance or tests.They are compatible and you can use them all in one project to build a powerful application. It’s no wonder that thousands of professionals use them in their daily work.
The article was created in collaboration by Michał Niciński, Frontend developer and Mateusz Grzesiukiewicz, Senior JavaScript developer at Boldare.
Share this article: