How to use JavaScript Classes? Three Different Ways
Programmers love JavaScript. They love it for its extremely flexible approach and the countless possibilities for creating the same mechanics in completely different ways. A perfect example of JavaScript flexibility is the way classes are defined in this language. How to use classes in JavaScript?
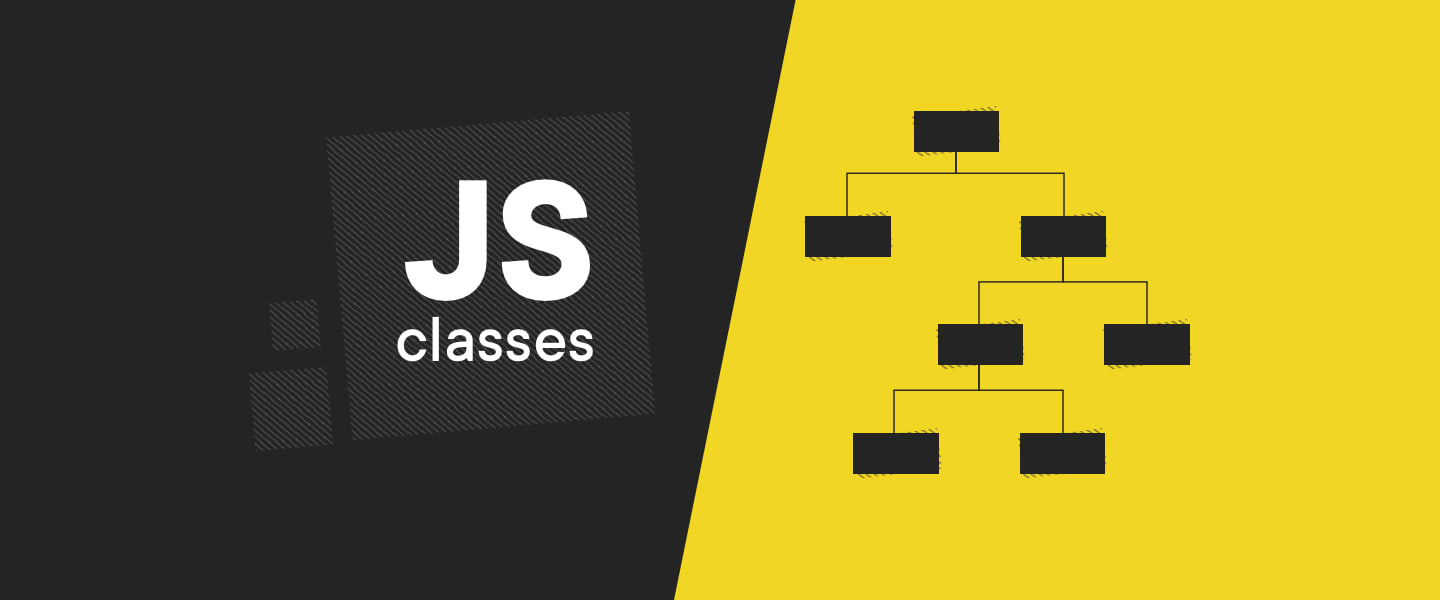
Table of contents
As you probably know, the definition of JavaScript class isn’t as obvious as in other popular languages like Java, C# or PHP. In this language, a class is unusual because this mechanism is working on prototypes.
Let’s focus on three types of classes for JavaScript to understand how you can use them. They are:
- prototype-based classes,
- singleton objects and object literals,
- ES6 classes.
Note: This article assumes that you have already basic knowledge about JavaScript and will follow some examples without detailed explanations.
Prototype-based classes
What is interesting in this case is that the class syntax is versatile. Let start with a simple prototype-based JavaScript class example. The example below presents a method defined internally. This solution can prevent pollution of the global namespace because all methods are defined within the constructor function.
function Person(name) {
this.name = name;
this.welcome = function() {
console.log(“Welcome “ + this.name);
};
}
The second example shows the method added to the prototype. In this case, the “welcome” method is not recreated every time when the new object is initializing. It’s not a popular solution but in some cases can be what we want to achieve.
function Person(name) {
this.name = name;
}
Person.prototype.welcome = function () {
console.log("Welcome " + this.name);
}
It’s time to present how to demonstrate an object using the Person constructor function, setting some properties and call methods.
const person = new Person("Bob");
person.welcome(); // Welcome Bob
Singleton objects and object literals
Let’s focus now on a method which allows you to use a function to define a singleton object in the JavaScript language. This seems really similar to the previous example but is more compact. In the below example, you can see that defining a function and invoking creation of the object is running at the same time.
const person = new function () {
this.name = "Bob";
this.welcome = function () {
console.log("Welcome " + this.name);
}
};
person.welcome(); // Welcome Bob
It’s little confusing because it has been used as an anonymous constructor function and we can’t add dynamic arguments. As a result, we have only one single instance of this class.
The other alternative includes the object literals syntax which allows immediate definition of singleton objects.
const person = {
name: "Bob",
welcome: function () {
console.log("Welcome " + this.name);
}
};
person.welcome(); // Welcome Bob
In this case, the instance already exists and is ready to use. It’s easy, right?
ES6 Classes
Let us take a look at what has been introduced in ES6 class methods. One of the new things is “syntactic sugar” for classes which is like the overlay on this mechanism.
Thereby, “syntactic sugar” means that the new features of the language are not really new because the mechanism is the same with improvements in syntax only.
Therefore, the class constructs allow defining prototype-based classes with clean syntax for programmers. Let’s work through an example to understand what they look like.
class Person {
constructor(name) {
this.name = name;
}
welcome() {
console.log("Welcome " + this.name);
}
}
const person = new Person("Bob");
person.welcome(); // Welcome Bob
It is essential in all these types of JavaScript classes to remember that:
- the class constructor can’t be called without a “new” operator,
- there cannot exist more than one constructor in the class
- during object creation, the first step is running the “constructor” method with a particular initial set of properties and values,
- if “constructor” does not exist in the class then what is generated is an empty function. Then you can execute other implemented methods on created objects,
- the code inside the class is automatically in strict mode.
The new class syntax also supports inheritance and static methods in a simple way. The inherited object contains all the properties and functions of its parent object. In addition, it allows the specification of its own set of unique properties and functions or the overwriting of existing properties and functions.
In consequence, inheritance helps us write cleaner code and re-purpose the parent object to save memory on repeating the object property and method definitions.
class Mechanic extends Person {
constructor(name) {
super(name);
}
fix() {
console.log(this.name + " fixed your car");
}
static clean() {
console.log("Static method");
}
}
Mechanic.clean(); // Static method
const mechanic = new Mechanic("John");
mechanic.welcome(); // Welcome John
mechanic.fix(); // John fixed your car
Let’s explain what’s new about the above code. We used the word static in the “clean” method which defines static methods. This type of method is mainly used to implement methods that belong to the class but are invoked without class initialization. That means that it can’t be invoked by the instance of the class. Furthermore, static methods can also be inherited and can be invoked from subclasses.
You’ll also notice a new word “super” in the constructor. The super keyword is used to access and call functions from an object’s parent. Additionally, you need to remember that the constructor can be used before the “this” keyword is used.
Summary
Let’s wrap this article up. By and large, we can’t say with a clear conscience that JavaScript classes exist because the whole mechanism is based on prototypes. Notwithstanding that, the flexibility of this language provides the developer with many different ways to achieve the desired results.
New versions of ECMAScript standards were introduced with more developer-friendly features. In consequence, this allows writing code in a more readable way. But on the other hand, it does not mean that we shouldn’t think about why and how some covers work in this way because that can be turn out to be important in some cases of usage.
Share this article: